Spiral Matrix II
Given a positive integer n
, generate an n x n
matrix
filled with elements from 1
to n2
in spiral order.
Example 1:
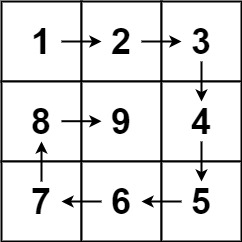
Input: n = 3 Output: [[1,2,3],[8,9,4],[7,6,5]]
Example 2:
Input: n = 1 Output: [[1]]
Constraints:
-
1 <= n <= 20
Solution:
class Solution {
public int[][] generateMatrix(int n) {
int[][] matrix = new int[n][n];
int top = 0, bottom = n - 1, left = 0, right = n
- 1;
int val = 1;
while (val <= n * n) {
for (int i = left; i <= right;
i++)
matrix[top][i] =
val++;
top++;
for (int i = top; i <= bottom;
i++)
matrix[i][right] =
val++;
right--;
for (int i = right; i >= left;
i--)
matrix[bottom][i] =
val++;
bottom--;
for (int i = bottom; i >= top;
i--)
matrix[i][left] =
val++;
left++;
}
return matrix;
}
}
Diagram in the problem statement clearly explains about matrix flow. Now, we
are going to code accordingly by following the above diagram.
1) First for-loop is used to fill top row.
2) Second for-loop is used to fill right column.
3) Third for-loop is used to fill bottom row.
4) Fourth for-loop is used to fill left column.
5) Repeat above steps till val <= n*n. For any n, it will be executed and
rotate spirally and fill entire matrix.
Comments
Post a Comment