Leftmost Column with at Least a One
A binary matrix means that all elements are
Given a row-sorted binary matrix binaryMatrix, return leftmost column index(0-indexed) with at least a
You can't access the Binary Matrix directly. You may only access the matrix using a
For custom testing purposes you're given the binary matrix
It is one of FACEBOOK INTERVIEW question as per Leetcode.
Example 1:



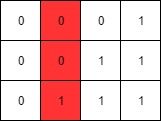
Constraints:
Try it on Leetcode
Here, each row is sorted in non - decreasing order(as per question).
1) We are getting number of rows, columns using dimensions() and store it as a List. It is having only m, n.
2) We are traversing from end of column and from start of the row. If there is any 1 then decrease the column and if there is any 0 increase row.
3) Repeat the step 2, until you reaching only one 1 in a column at leftmost.(we are starting at right and reaching left).
0
or 1
. For each individual row of the matrix, this row is sorted in non-decreasing order.Given a row-sorted binary matrix binaryMatrix, return leftmost column index(0-indexed) with at least a
1
in it. If such index doesn't exist, return -1
.You can't access the Binary Matrix directly. You may only access the matrix using a
BinaryMatrix
interface:BinaryMatrix.get(x, y)
returns the element of the matrix at index(x, y)
(0-indexed).BinaryMatrix.dimensions()
returns a list of 2 elements[n, m]
, which means the matrix isn * m
.
1000
calls to BinaryMatrix.get
will be judged Wrong Answer. Also, any solutions that attempt to circumvent the judge will result in disqualification.For custom testing purposes you're given the binary matrix
mat
as input in the following four examples. You will not have access the binary matrix directly.It is one of FACEBOOK INTERVIEW question as per Leetcode.
Example 1:

Input: mat = [[0,0],[1,1]] Output: 0Example 2:

Input: mat = [[0,0],[0,1]] Output: 1Example 3:

Input: mat = [[0,0],[0,0]] Output: -1Example 4:
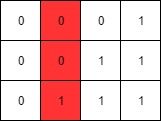
Input: mat = [[0,0,0,1],[0,0,1,1],[0,1,1,1]] Output: 1
Constraints:
1 <= mat.length, mat[i].length <= 100
mat[i][j]
is either0
or1
.mat[i]
is sorted in a non-decreasing way.
/**
* // This is the BinaryMatrix's API interface.
* // You should not implement it, or speculate about its implementation
* interface BinaryMatrix {
* public int get(int x, int y) {}
* public List<Integer> dimensions {}
* };
*/
class Solution {
public int leftMostColumnWithOne(BinaryMatrix binaryMatrix) {
List<Integer>dimension = binaryMatrix.dimensions();
int row = dimension.get(0), m=0, n=dimension.get(1)-1;
int result = -1;
while(m<row && n>=0){
if(binaryMatrix.get(m, n) == 1){
result = n;
n--;
}
else{
m++;
}
}
return result;
}
}
Try it on Leetcode
Here, each row is sorted in non - decreasing order(as per question).
1) We are getting number of rows, columns using dimensions() and store it as a List. It is having only m, n.
2) We are traversing from end of column and from start of the row. If there is any 1 then decrease the column and if there is any 0 increase row.
3) Repeat the step 2, until you reaching only one 1 in a column at leftmost.(we are starting at right and reaching left).
Comments
Post a Comment